1. What is Compilation?
Compilation is the process of converting the entire source code (written in a high-level programming language) into machine code (binary code) before execution. The result of this process is an executable file that the computer can run directly.
How It Works:
- The source code is passed to the compiler.
- The compiler translates it into machine code.
- The machine code is stored in an executable file.
- The executable file is run on the system.
Key Features of Compilation:
- Pre-execution Translation: The whole program is translated before it is executed.
- Error Detection: Errors are reported after the entire source code is scanned.
- Faster Execution: Once compiled, the program runs very quickly.
- Example Languages: C, C++, Java (partially).
2. What is Interpretation?
Interpretation is the process of translating and executing the source code line by line. No separate executable file is created; the program runs directly through an interpreter.
How It Works:
- The source code is given to the interpreter.
- The interpreter reads one line of code, translates it into machine code, and executes it immediately.
- This process repeats for every line of the program.
Key Features of Interpretation:
- Line-by-Line Execution: The program is translated and executed simultaneously.
- Error Reporting: Errors are reported immediately when encountered.
- Slower Execution: The program takes longer to execute since translation happens during runtime.
- Example Languages: Python, JavaScript, PHP.
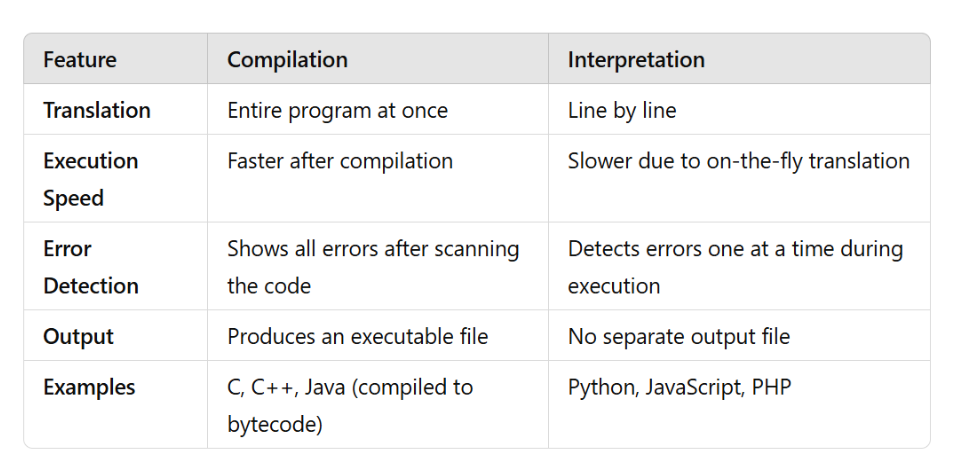
3. Compilation + Interpretation in Modern Languages
Some modern languages, like Java, use a combination of compilation and interpretation.
- The Java compiler translates source code into bytecode (intermediate code).
- The Java Virtual Machine (JVM) interprets and executes the bytecode.